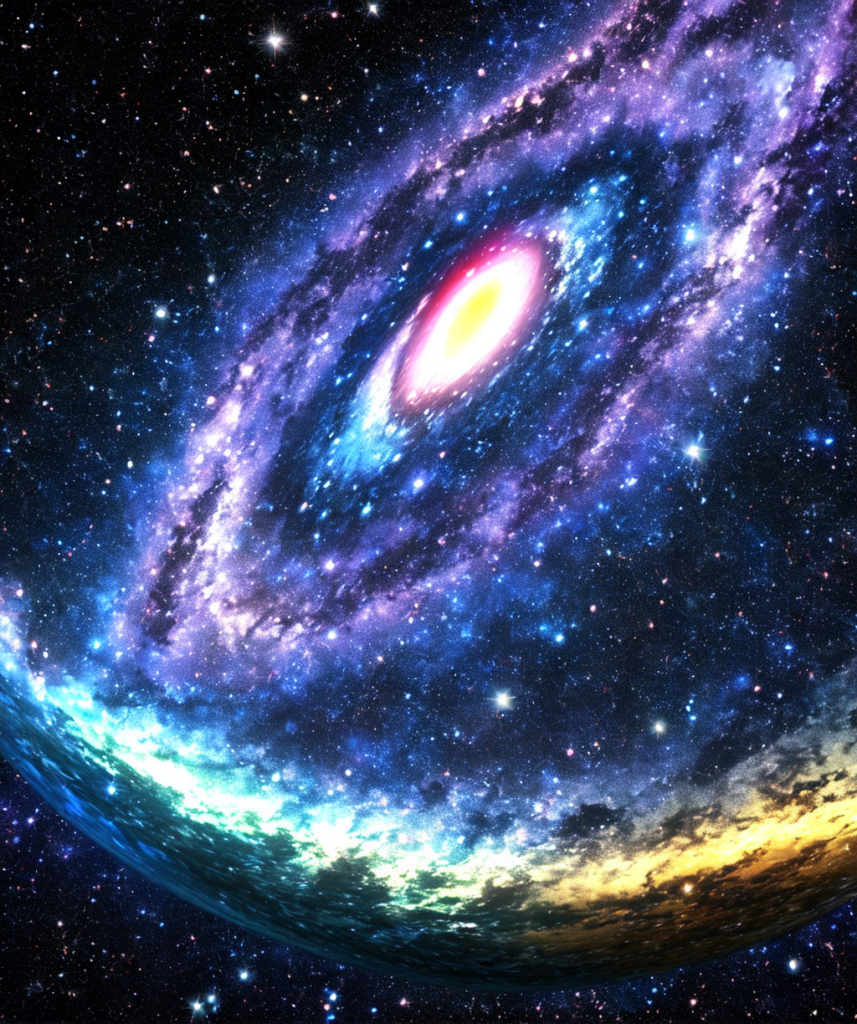
Python
So, I’ve been learning very slowly a bit of Python.
And Between a friend and Chat GPT (They made what I’m about to share, this is something simple I’ve been using to learn.
This is a very simple text movement thing, there’s absolutely nothing really “heavy” about it. But it’s a pretty great tool to figure out how to get things to work together I’ve found.
#!/usr/bin/env python
# Adventure map of the game
adventure_map = [
["central hallway", "A hallway connecting all the rooms", [1, 3, 2, 4]],
["living room", "A cozy room with a fireplace", [None, 0, None, None]],
["kitchen", "A room with cooking appliances and a dining table, there's a larder to the south", [None, 5, None, 0]],
["bedroom", "A peaceful room with a bed and a closet to the west", [0, None, None, 6]],
["bathroom", "A small room with a shower and toilet", [None, None, 0, None]],
["larder","a storage space for food, the kitchen is north.",[2,None, None, None]],
["closet","a place for storing clothing, the bedroom is east.",[0, None, 3, None]]
]
# Starting room
current_room = 0
NORTH=0
SOUTH=1
EAST=2
WEST=3
while True:
room_info=adventure_map[current_room]
room_name=room_info[0]
room_description=room_info[1]
directions_available=room_info[2]
print(f"--{room_name}--\n {room_description}")
raw_direction = input("Which way would you like to go? (north, south, east, west) ")
direction=raw_direction.lower()
if direction == "north":
next_room = directions_available[NORTH]
elif direction == "south":
next_room = directions_available[SOUTH]
elif direction == "east":
next_room = directions_available[EAST]
elif direction == "west":
next_room = directions_available[WEST]
else:
print("Invalid direction.")
continue
if next_room is not None:
current_room = next_room
else:
print("You can't go that way.")